Ionic 5 image slider with example
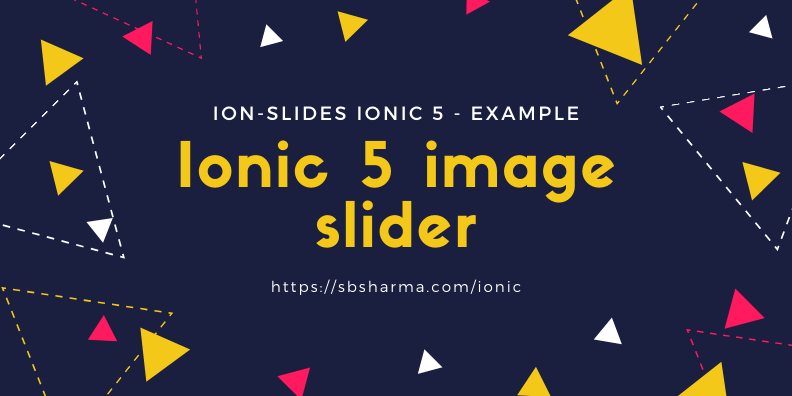
Today we will learn about ionic image slider with example. I will try to explain every important aspect of Ion-slides. Ionic usage swiper js library for slides, so we can use most of the available options of swiper js with ion-slides.
Read also : How to add custom component to the ionic 5
Let’s start with basic example
Basic example of Ion-slides
The basic example of ion-slides teaches us how we can start with ion-slides. The minimum options passed to the slides. Here we can see the basic syntax of slides, we can pass the images to these slides.
export class imageSlider { // Optional parameters to pass to the swiper instance. // See http://idangero.us/swiper/api/ for valid options. slideOpts = { initialSlide: 1, speed: 400 }; constructor() {} }
Let’s create the template for this image slider.
<ion-content> <ion-slides [options]="slideOpts"> <ion-slide> <img src=”assets/imgs/nature-beauty.png” alt=”slide-1” /> </ion-slide> <ion-slide> <img src=”assets/imgs/playing-kids.png” alt=”slide-2” /> </ion-slide> <ion-slide> <img src=”assets/imgs/water-drops.png” alt=”slide-3” /> </ion-slide> </ion-slides> </ion-content>
Loop the slides – example
What happens when all slides finish to scroll? We only can slide back in reverse order. But if we want to keep sliding forward we have to pass the loop true to the slide options. It will allow us to keep sliding without caring about the end of slides. Basically it will loop all the slides again and again.
slideOpts = { initialSlide: 1, speed: 400, loop: true };
Auto Play slides – example
Most of the time we want to play our slides automatically with some time intervals. This is called autoplay and we can achieve this by doing the following.
slideOpts = { initialSlide: 1, speed: 400, loop: true, autoplay: { delay: 4000 } };
Here the delay is in milliseconds, so 4000 means 4 seconds.
Auto Play slides in reverse direction- example
If you want to play your slides in reverse order you can use reverseDirection true and pass to the autoplay options.
slideOpts = { initialSlide: 1, speed: 400, loop: true, autoplay: { delay: 4000, reverseDirection: true } };
Keep autoplay after user interaction
When the user clicks on the slide or drag the slide it will stop autoplay of the slides. We can change this behaviour by passing the parameter disableOnInteraction as false to autoplay options.
slideOpts = { initialSlide: 1, speed: 400, loop: true, autoplay: { delay: 4000, disableOnInteraction: false, } };
Stop on last slide
Sometimes we want to play our slides only once and when all slides finish we want to stop the slider. Keep in mind that a loop should not be added here if we want to use the stopOnLastSlide option. We can achieve this by following example
slideOpts = { initialSlide: 1, speed: 400, autoplay: { delay: 4000, disableOnInteraction: false, stopOnLastSlide: true } };
Enable Pager for the slides
Just pass the pager to the ion-slides and it will add the numbering or dot to the bottom of the slides.
<ion-slides pager="true" [options]="slideOpts">
Clickable pagination
Clickable pagination means we can change the slide by clicking on the pagination. Like if we have bullets as a pagination type, we can change our slides by clicking on one of the bullets.
slideOpts = { initialSlide: 0, speed: 400, pagination : { el: '.swiper-pagination', clickable: true } };
Change pagination type
By default pagination type is bullets. We can change it to ‘bullets’ | ‘fraction’ | ‘progressbar’ | ‘custom’.
slideOpts = { initialSlide: 0, speed: 400, pagination : { el: '.swiper-pagination', type: 'progressbar' , clickable: true } }
Change progressbar color
So, I want to change the progress bar color. I have tried by adding class to our tab1.scss file with background color red for the progress bar. But it didn’t work. Then I tried the solution below which actually works very well.
slideOpts = { initialSlide: 0, speed: 400, loop: true, autoplay: { delay: 2000 }, pagination : { el: '.swiper-pagination', clickable: true, type: 'progressbar', progressbarFillClass: 'swiper-pagination-progressbar-fill', renderProgressbar: function (progressbarFillClass) { return '<span class="' + progressbarFillClass + '" style="background: red"></span>'; } } }
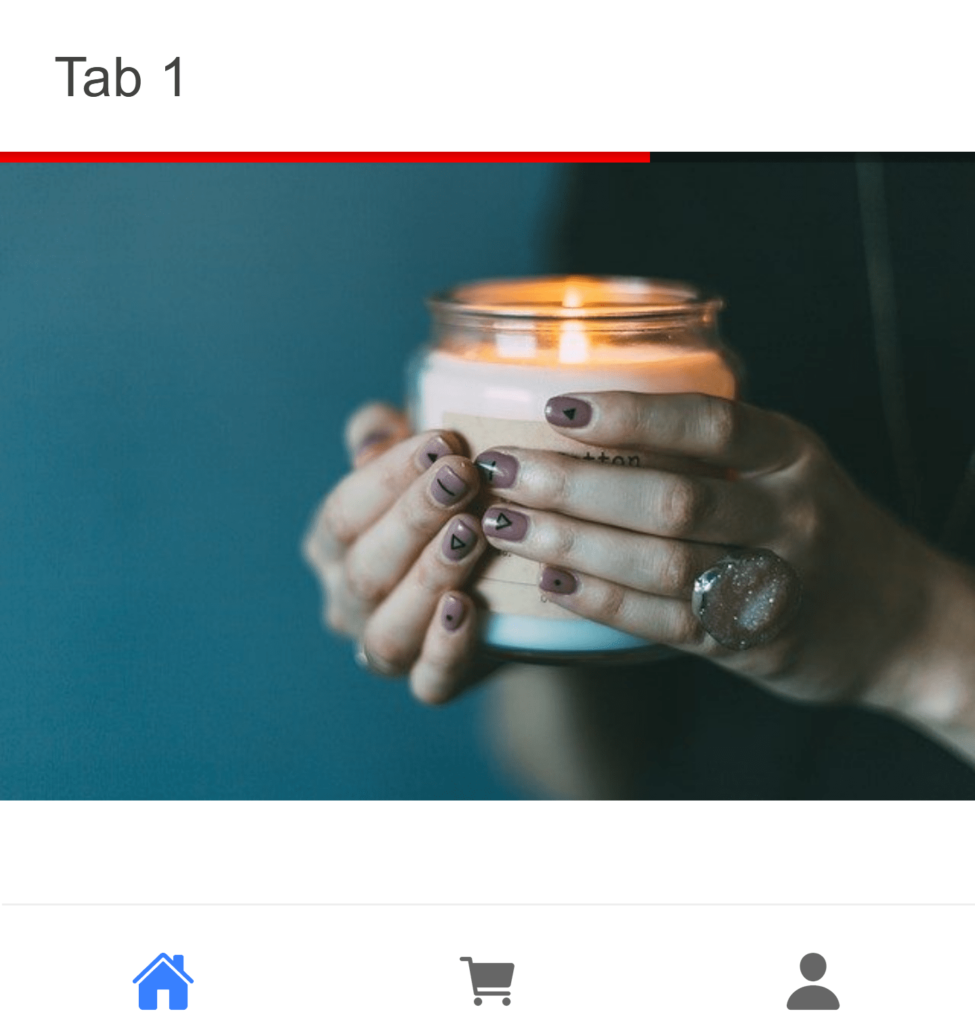
Same way we have “renderFraction” for pagination type “fraction” and “renderBullet” for “bullets”. We can also customize these elements.
Slides per view and centered slides
slideOpts = { slidesPerView: 1.5, spaceBetween: 5, speed: 400, loop: true, centeredSlides: true, autoplay: { delay: 2000 }, }
Slides per view defines how many slides can view per slide. Like we have set it to 1.5 means 1 slide full and the other two will be 25% each because it is also set to centered.
If we remove the centered slides option then it will take one slide full and next half.
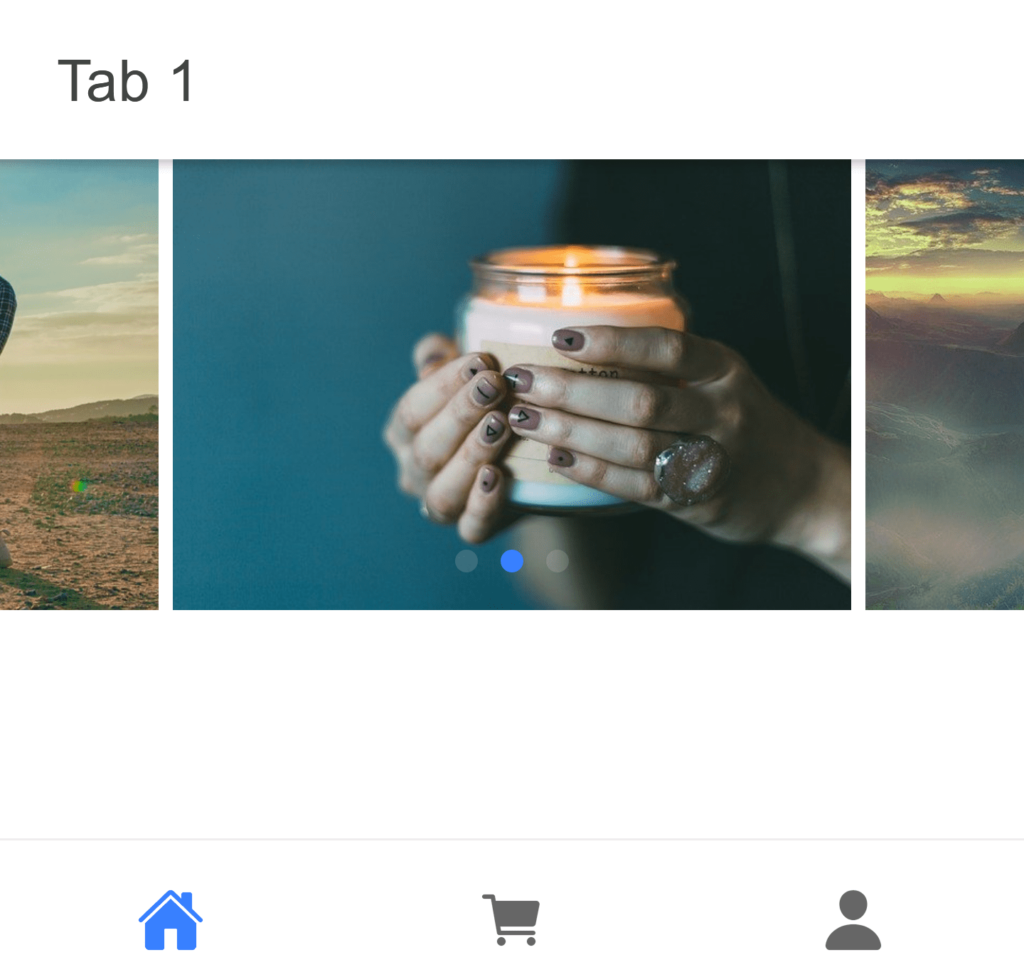
That’s all. Yes, there are lot more but we will discuss them later. Above are the very common needs of ionic image slider.
Hope this article will useful for you.