Laravel 9 social login with step by step guide – Example
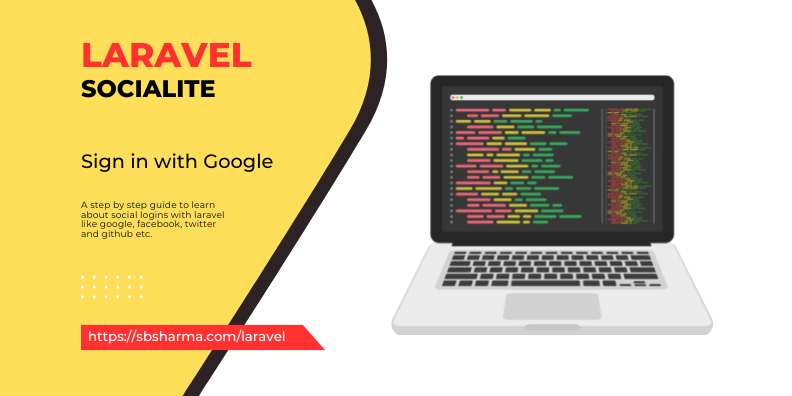
I think almost all applications come with social login these days, and it makes sense to use google, facebook, github accounts to make our login process easier.
Laravel comes with several built-in features that make web development easier, including Socialite. Socialite is a Laravel package that provides an easy way to use social logins to authenticate users. We can use various OAuth providers like Google login, Facebook login, Twitter login, github login, and more.
In this tutorial, we will show you how to implement Google authentication using Laravel 9 Socialite. We will walk you through the process step by step and provide you with all the necessary code examples.
Step 1: Ensure Laravel 9 prerequisites
Before we start, make sure you have the following installed on your computer:
- PHP version 7.4 or higher
- Composer
- Laravel 9
Step 2: Create a Google OAuth Application
To use Google authentication with Laravel 9 Socialite, you first need to create a Google OAuth application. Follow the steps below to create your Google OAuth application:
- Go to the Google Cloud Console and sign in to your account.
- Create a new project by clicking on the “Select a Project” dropdown at the top of the page and then clicking on the “New Project” button.
- Enter a name for your project and click on the “Create” button.
- Once your project is created, click on the “APIs & Services” tab on the left sidebar and then click on the “Credentials” option.
- Click on the “Create Credentials” button and select “OAuth client ID“.
- Choose “Web application” as the application type and enter a name for your OAuth client.
- Under “Authorized JavaScript origins“, enter your domain name (e.g. http://localhost:8000).
- Under “Authorized redirect URIs“, enter the URL for the callback route (e.g. http://localhost:8000/callback/google).
- Click on the “Create” button to create your OAuth client ID.
Step 3: Install Laravel 9 Socialite
To install Laravel 9 Socialite, run the following command in your terminal:
composer require laravel/socialite
Step 4: Add Google OAuth Credentials to .env
After you have installed Laravel 9 Socialite, you need to add your Google OAuth credentials to your .env
file. Open your .env
file and add the following lines:
GOOGLE_CLIENT_ID=your_client_id GOOGLE_CLIENT_SECRET=your_client_secret GOOGLE_REDIRECT=http://localhost:8000/callback/google
Replace your_client_id
and your_client_secret
with the client ID and secret from your Google OAuth client.
Step 5: Create Routes
Now that we have installed Laravel 9 Socialite and added our Google OAuth credentials to our .env
file, we need to create routes for our authentication flow. Open your routes/web.php
file and add the following code:
use Illuminate\Support\Facades\Route; use App\Http\Controllers\Auth\LoginController; Route::get('/login/google', [LoginController::class, 'redirectToGoogle'])->name('login.google'); Route::get('/callback/google', [LoginController::class, 'handleGoogleCallback']);
These routes will redirect the user to Google for authentication and handle the callback from Google.
Step 6: Create LoginController
Next, we need to create a LoginController
that will handle the authentication flow. Create a new file called LoginController.php
in the app/Http/Controllers/Auth
directory and add the following
<?php namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Support\Facades\Auth; use Laravel\Socialite\Facades\Socialite; use App\Models\User; class LoginController extends Controller { public function redirectToGoogle() { return Socialite::driver('google')->redirect(); } public function handleGoogleCallback() { $user = Socialite::driver('google')->user(); $existingUser = User::where('email', $user->getEmail())->first(); if ($existingUser) { Auth::login($existingUser, true); } else { $newUser = new User; $newUser->name = $user->getName(); $newUser->email = $user->getEmail(); $newUser->google_id = $user->getId(); $newUser->save(); Auth::login($newUser, true); } return redirect('/dashboard'); } }
Step 7: Create Views
Finally, we need to create views for our authentication flow. Create a new file called login.blade.php
in the resources/views/auth
directory and add the following code:
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header">{{ __('Login') }}</div> <div class="card-body"> <a href="{{ route('login.google') }}" class="btn btn-primary">{{ __('Login with Google') }}</a> </div> </div> </div> </div> </div> @endsection
Conclusion
In this tutorial, we have shown you how to implement Google authentication using Laravel 9 Socialite. We walked you through the process step by step and provided you with all the necessary code examples.
With this comprehensive guide, you will be able to implement Google authentication using Laravel 9 Socialite easily. We hope that this tutorial was helpful to you. Good luck!
Wonderful website. Lots of useful information here. I am sending it to a few pals ans also sharing in delicious. And of course, thanks to your effort!