Retrieving unique Values from two array – JavaScript
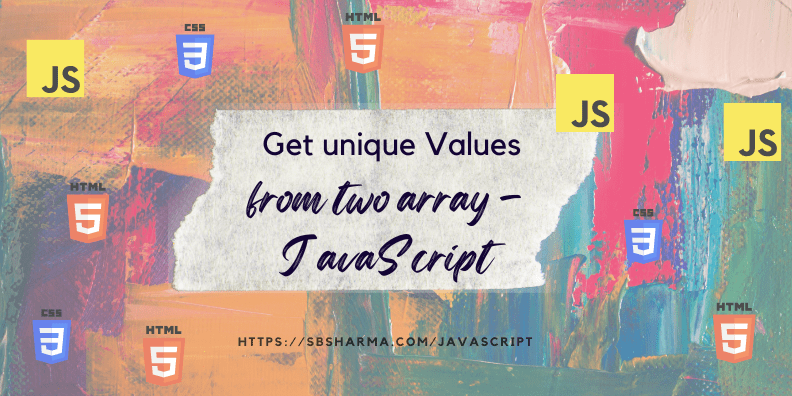
Arrays are a fundamental data structure in JavaScript, and comparing arrays is a common task in many programming scenarios. In this tutorial, we will explore how to compare two arrays and extract the matching unique values using JavaScript. By the end of this article, you’ll have a clear understanding of the process and be able to apply it to your own projects.
Let’s consider two arrays, array1
and array2
, as our starting point. Our goal is to find the matching unique values between these arrays. In other words, we want to extract the values that exist in both arrays while eliminating any duplicates.
Here’s a step-by-step guide to retrieve the unique values from a JavaScript array:
Step 1: Define the arrays
Start by defining two arrays that you want to compare. For the purpose of this tutorial, let’s call them array1
and array2
.
const array1 = [1, 2, 3, 4, 5]; const array2 = [4, 5, 6, 7, 8];
Step 2: Create a function to compare arrays
Next, create a function that will compare the arrays and return the matching unique values. Let’s name this function getMatchingUniqueValues
.
function getMatchingUniqueValues(array1, array2) { // Code goes here }
Step 3: Iterate over the arrays
Inside the getMatchingUniqueValues
function, start by iterating over one of the arrays. In this case, we’ll iterate over array1
using the forEach
method.
function getMatchingUniqueValues(array1, array2) { const matchingValues = []; array1.forEach((value) => { // Code goes here }); return matchingValues; }
Step 4: Check for matching values
Within the forEach
loop, use the includes method to check if the current value exists in array2
. If it does, push it to the matchingValues
array.
function getMatchingUniqueValues(array1, array2) { const matchingValues = []; array1.forEach((value) => { if (array2.includes(value)) { matchingValues.push(value); } }); return matchingValues; }
Step 5: Filter out duplicate values
To ensure that the resulting array only contains unique values, you can use the filter method and the indexOf
method to remove any duplicates.
function getMatchingUniqueValues(array1, array2) { const matchingValues = []; array1.forEach((value) => { if (array2.includes(value) && matchingValues.indexOf(value) === -1) { matchingValues.push(value); } }); return matchingValues; }
Step 6: Test the function
Finally, you can test the function by calling it with the defined arrays and log the output.
const array1 = [1, 2, 3, 4, 5]; const array2 = [4, 5, 6, 7, 8]; const result = getMatchingUniqueValues(array1, array2); console.log(result);
When you run the code, the console will display the matching unique values between array1
and array2
.
Conclusion:
In this tutorial, we’ve explored how to compare arrays and retrieve the matching unique values using JavaScript. By following the step-by-step guide, you’ve learned how to create a function that can tackle this common task. Remember, array manipulation is an essential skill in JavaScript, and this knowledge will empower you to handle array comparisons effectively in your own projects.
Additional Resources:
- MDN Web Docs: Array.prototype.includes
- MDN Web Docs: Array.prototype.filter