Most asked 20 JavaScript interview questions for beginners with answers & examples
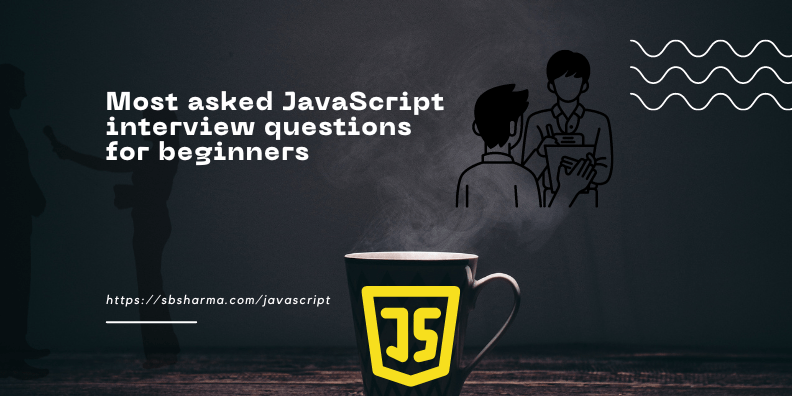
Most Asked JavaScript Interview Questions for beginners
In this article, we will reveal the top 20 JavaScript interview questions for beginners that will keep you on the edge of your seat during your journey to becoming a JavaScript expert.
These questions are carefully curated, often asked in interviews at prestigious companies, and designed to test your fundamental understanding of the language.
So, let’s get started and unlock the secrets of JavaScript!
1. Difference between JavaScript and Java?
Answer: JavaScript is a high-level, interpreted programming language primarily used for front-end web development. It is unrelated to Java, which is a separate, fully-fledged programming language used for a variety of applications.
2. What are the different data types in JavaScript?
Answer: JavaScript supports various data types, including array, numbers, strings, boolean, null, undefined, objects, and symbols.
3. Explain the difference between let
, const
, and var
in variable declaration.
Answer: let
and const
are block-scoped, while var
is function-scoped. Additionally, variables declared with const
cannot be reassigned once defined, whereas let
and var
can be.
let’s demonstrate functional scope and block-level scope for var
, let
, and const
using examples:
Functional scope means that a variable declared with var
is accessible throughout the entire function where it is declared, regardless of the block it’s in. This can sometimes lead to unintended consequences if not handled properly.
function ScopeExample() { if (true) { var nameVar = "Alice"; // 'var' is function-scoped let nameLet = "Bob"; // 'let' is block-scoped const nameConst = "Charlie"; // 'const' is block-scoped console.log(nameVar); // Output: Alice console.log(nameLet); // Output: Bob console.log(nameConst); // Output: Charlie } console.log(nameVar); // Output: Alice (var is accessible outside the if block) // console.log(nameLet); // Error: nameLet is not defined (let is block-scoped) // console.log(nameConst); // Error: nameConst is not defined (const is block-scoped) } ScopeExample();
In this example, nameVar
declared with var
is accessible outside the if
block because of its functional scope. However, both nameLet
and nameConst
, declared with let
and const
, respectively, are not accessible outside the if
block because they are block-scoped.
Using block-scoped variables (let
and const
) helps avoid unintended variable hoisting and potential bugs that can arise due to functional scoping.
4. What is hoisting in JavaScript?
Answer: Hoisting in JavaScript is a behavior where the compiler moves variable and function declarations to the top of their containing scope.
This behavior allows you to utilize variables and invoke functions before their actual declaration in the code. It’s important to note that hoisting only affects declarations, not the initialization of variables or functions.
Let’s demonstrate hoisting with a suitable example:
// Example 1 - Variable Hoisting console.log(x); // Output: undefined var x = 10; // The above code is equivalent to: // var x; // console.log(x); // x = 10; // Example 2 - Function Hoisting foo(); // Output: "Hello, I am foo!" function foo() { console.log("Hello, I am foo!"); } // The above code is equivalent to: // function foo() { // console.log("Hello, I am foo!"); // } // foo(); // Example 3 - Function Expression Hoisting bar(); // Output: TypeError: bar is not a function var bar = function() { console.log("Hello, I am bar!"); }; // The above code is equivalent to: // var bar; // bar(); // TypeError: bar is not a function // bar = function() { // console.log("Hello, I am bar!"); // };
To avoid confusion and improve code readability, it is recommended to always declare variables and functions before using them. Understanding hoisting can help you be aware of how JavaScript behaves in such scenarios.
5. Explain the concept of “closures” in JavaScript.
Answer: Closures are functions that have access to variables from their outer (enclosing) scope, even after the outer function has finished executing.
Let’s demonstrate closures with an example:
function outerFunction(x) { // This inner function is a closure because it "closes over" the variable `x`. function innerFunction(y) { return x + y; } return innerFunction; } // Create closures by calling the outer function with different arguments. const closure1 = outerFunction(5); const closure2 = outerFunction(10); // Use the closures to perform operations. console.log(closure1(3)); // Output: 8 (5 + 3) console.log(closure2(7)); // Output: 17 (10 + 7)
Closures are powerful because they allow functions to have private variables and create a sort of “encapsulation” in JavaScript. People commonly use them in scenarios such as creating factory functions, keeping track of state across multiple function calls, and implementing module patterns to establish private members in objects.
6. How do you check the data type and equality of two variables in JavaScript?
Answer: To check the data type, use the typeof
operator. For equality, use ===
(strict equality) and ==
(loose equality).
7. What is the purpose of the this
keyword in JavaScript?
Answer: The this
keyword pertains to the current object context and individuals use it to access the object’s properties and methods within a function.
8. Explain the event bubbling and event capturing in JavaScript.
Answer: This is one of the most asked JavaScript interview questions for beginners. Event bubbling is when an event triggers on a nested element and then propagates up through its ancestors. Event capturing is the reverse, where the event is captured from the topmost ancestor down to the target element. We have already discussed this approach in the previous article.
9. How do you create and use a JavaScript class?
Answer: JavaScript uses prototype inheritance rather than classes, but ES6 introduced the `class` syntax to mimic class-based inheritance. Example:
class Animal { constructor(name) { this.name = name; } speak() { console.log(this.name + ' makes a noise.'); } } const dog = new Animal('Dog'); dog.speak(); // Output: Dog makes a noise.
10. Explain the concept of asynchronous programming in JavaScript.
Answer: Asynchronous programming allows non-blocking execution of code, enabling tasks like AJAX requests and timeouts without freezing the main thread.
11. How do you handle errors in JavaScript using try…catch?
Answer: The `try…catch` statement allows you to handle exceptions and errors gracefully by providing a fallback plan when an error occurs.
try { // Code that might throw an error } catch (error) { // Handle the error here }
12. What is the purpose of the `use strict` directive in JavaScript?
Answer: The "use strict"
directive enforces a stricter set of rules and better error handling in JavaScript, reducing silent errors and improving code quality.
13. Explain the concept of promises in JavaScript.
Answer: Promises are objects that represent the eventual completion (or failure) of an asynchronous operation, providing a cleaner way to handle callbacks.
const fetchData = () => { return new Promise((resolve, reject) => { // Asynchronous operation if (dataReceived) { resolve(data); } else { reject("Error: Data not received"); } }); }; fetchData() .then((data) => console.log(data)) .catch((error) => console.error(error));
14. What is the Event Loop in JavaScript?
Answer: The Event Loop is a mechanism in JavaScript that handles asynchronous tasks and callbacks, ensuring smooth execution by managing the callback queue and the call stack.
15. How do you perform object de-structuring in JavaScript?
Answer: Object de-structuring allows you to extract properties from an object and assign them to variables in a concise manner.
const person = { name: 'John', age: 30, gender: 'Male' }; const { name, age } = person; console.log(name, age); // Output: John 30
16. Explain the difference between `==` and `===` in JavaScript.
Answer: ==
performs type coercion, while ===
enforces strict equality, meaning the operands must have the same value and data type.
17. What are Arrow functions in JavaScript, and how are they different from regular functions?
Answer: Arrow functions are a shorthand syntax for writing functions in JavaScript, and they don’t bind their own this
value.
// Regular function function add(a, b) { return a + b; } // Arrow function const add = (a, b) => a + b;
18. How do you iterate over objects and arrays in JavaScript?
Answer: For arrays, you can use methods like forEach
, map
, filter
, etc. For objects, you can use for...in
loop or Object.keys()
/ Object.values()
.
const numbers = [1, 2, 3]; numbers.forEach((num) => console.log(num)); // Output: 1 2 3 const person = { name: 'Alice', age: 25 }; for (const key in person) { console.log(key, person[key]); // Output: name Alice, age 25 }
19. What is the difference between synchronous and asynchronous code execution?
Answer: Synchronous code executes line by line, blocking further execution until the current operation completes. Asynchronous code allows other tasks to run while waiting for certain operations to finish.
20. How do you handle CORS issues in JavaScript?
Answer: Cross-Origin Resource Sharing (CORS) issues can be handled by configuring the server to include appropriate CORS headers, allowing requests from specific domains.
Conclusion:
By understanding these fundamental concepts about JavaScript interview questions for beginners, you are now well-prepared to conquer JavaScript interviews at top companies. Keep exploring, honing your skills, and unraveling the mysteries of this versatile language.
Happy coding!