Add Extra fields in the default registration form – Laravel [Example + Source code]
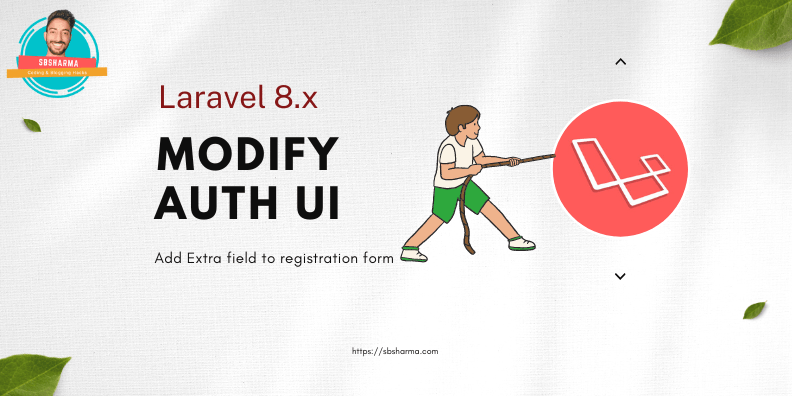
When we talk about the default laravel registration form, we might need to add some more fields in this registration form. Like address, mobile number, skill or anything. Adding extra field to the registration form laravel is very easy, let’s see with proper example.
I am going to add security questions in this registration form. Because I’ll continue this tutorial to another post, where we will discuss how to use these security questions with the normal login process.
Install the Laravel
To install the laravel run the below command.
composer create-project --prefer-dist laravel/laravel:^8.0 TestAuthUI
This command will set up your laravel 8.x project. If you have any issue check the laravel official website for installation process.
Go to the project directory and run the below command to install the auth UI.
Let’s install Laravel Auth UI
Following commands will set up the auth UI to our project.
composer require laravel/ui.*
php artisan ui bootstrap --auth
Then we need to install and run npm to compile our ui javascript.
npm install
npm run dev
npx mix
Create the database
When we finish the auth UI installation then we get the auth migrations like users, password reset tables etc. we need to create one more migration to add columns to users table i.e. security question.
php artisan make:migration add_col_to_users_table
Put the string column to the up method and down method to drop the same column in case of reverse migration.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class AddColToUsersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::table('users', function (Blueprint $table) { $table->string('security_question')->after('email'); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::table('users', function (Blueprint $table) { $table->dropColumn('security_question'); }); } }
Migrate user auth database
Migrate the database with simply below command.
php artisan migrate
The above command will set up the auth tables to the database and we are ready to add an extra field in the registration form ui.
Registration form UI
Go to the resources > views > auth > register blade file. Open it, and write down the code below (don’t copy-paste the code 😋).
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header">{{ __('Register') }}</div> <div class="card-body"> <form method="POST" action="{{ route('register') }}"> @csrf <div class="row mb-3"> <label for="name" class="col-md-4 col-form-label text-md-end">{{ __('Name') }}</label> <div class="col-md-6"> <input id="name" type="text" class="form-control @error('name') is-invalid @enderror" name="name" value="{{ old('name') }}" required autocomplete="name" autofocus> @error('name') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <label for="email" class="col-md-4 col-form-label text-md-end">{{ __('Email Address') }}</label> <div class="col-md-6"> <input id="email" type="email" class="form-control @error('email') is-invalid @enderror" name="email" value="{{ old('email') }}" required autocomplete="email"> @error('email') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <label for="security_question" class="col-md-4 col-form-label text-md-end">{{ __('Who is your best friend?') }}</label> <div class="col-md-6"> <input id="security_question" type="text" class="form-control @error('security_question') is-invalid @enderror" name="security_question" value="{{ old('security_question') }}" required> @error('security_question') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <label for="password" class="col-md-4 col-form-label text-md-end">{{ __('Password') }}</label> <div class="col-md-6"> <input id="password" type="password" class="form-control @error('password') is-invalid @enderror" name="password" required autocomplete="new-password"> @error('password') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <label for="password-confirm" class="col-md-4 col-form-label text-md-end">{{ __('Confirm Password') }}</label> <div class="col-md-6"> <input id="password-confirm" type="password" class="form-control" name="password_confirmation" required autocomplete="new-password"> </div> </div> <div class="row mb-0"> <div class="col-md-6 offset-md-4"> <button type="submit" class="btn btn-primary"> {{ __('Register') }} </button> </div> </div> </form> </div> </div> </div> </div> </div> @endsection
As you can see, we have added the security question field in the registration form after the email field. That’s it, simple?
Registration Model
After adding the extra field to the registration form ui, we also need to include this extra field to the registration model via fillable property.
<?php namespace App\Models; use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use Laravel\Sanctum\HasApiTokens; class User extends Authenticatable { use HasApiTokens, HasFactory, Notifiable; /** * The attributes that are mass assignable. * * @var array<int, string> */ protected $fillable = [ 'name', 'email', 'security_question', 'password', ]; /** * The attributes that should be hidden for serialization. * * @var array<int, string> */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast. * * @var array<string, string> */ protected $casts = [ 'email_verified_at' => 'datetime', ]; }
Register controller
Put some validators for this extra field in the registration controller validators. Like below,
protected function validator(array $data) { return Validator::make($data, [ 'name' => ['required', 'string', 'max:255'], 'email' => ['required', 'string', 'email', 'max:255', 'unique:users'], 'security_question' => ['required', 'string', 'min:5', 'max:255'], 'password' => ['required', 'string', 'min:8', 'confirmed'], ]); }
Then add the security question in the array to store to the database.
protected function create(array $data) { return User::create([ 'name' => $data['name'], 'email' => $data['email'], 'security_question' => $data['security_question'], 'password' => Hash::make($data['password']), ]); }
Now, everything is ready to store security questions as an extra field in the registration form.
Serve the project to the browser
Now, run the below command to serve our project to the browser.
php artisan serve
Now, open the registration form by clicking on the registration link given in the right top corner.
Then fill the form and hit the submit button. Let’s check in the registration controller for the available data to store.
To test this just put the dd()
there in the app/http/controllers/auth/RegisterController
create method.
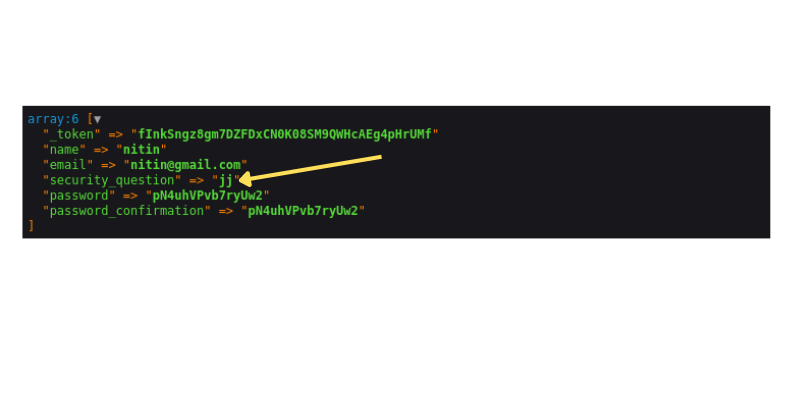
Conclusion
Now, you can easily add extra fields in the default registration form of laravel 8.x. You Only need to modify these things in the same order: registration form, Database migration, related model, and controller.