What is async and await in JavaScript for beginners?
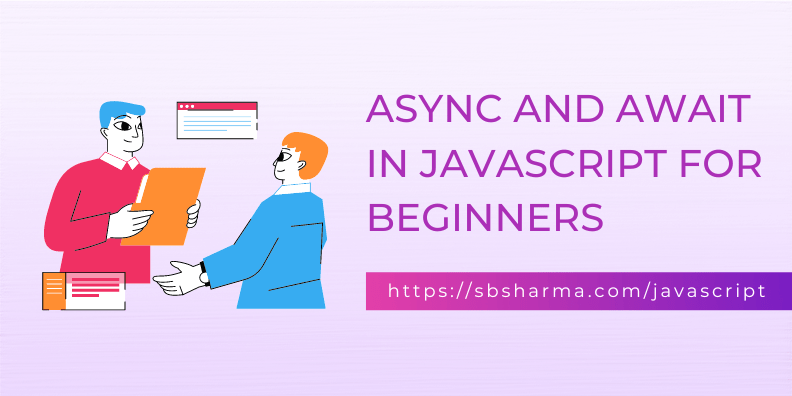
JavaScript has evolved significantly over the years, introducing new features and syntactic sugar to make asynchronous programming more manageable. One such powerful addition is the introduction of async and await, which simplify the handling of asynchronous operations.
We’ll dive straight into practical examples to understand the real-world benefits of async and await.
1. Explain Asynchronous JavaScript
To explain the async and await in javascript, we need to explain the asynchronous operations first.
In JavaScript, asynchronous operations are tasks that do not occur immediately but are scheduled to run at a later time. These operations could include making API calls, reading files, or executing tasks that require time.
Traditionally, developers have used callbacks and promises to handle asynchronous code. While effective, these methods can lead to “callback hell” or complex promise chaining.
2. Introducing async and await for beginners
The async and await keywords were introduced in ECMAScript 2017 (ES8) to simplify the handling of asynchronous operations. By combining them, developers can write asynchronous code in a more synchronous-looking style, making it easier to understand and maintain.
Let’s see the difference between async and await keywords in javascript.
The async
keyword is used before a function declaration to indicate that the function will contain asynchronous operations. This declaration implicitly returns a Promise, allowing you to use .then()
and .catch()
methods.
The await
keyword can only be used inside an async function. It pauses the execution of the function until the promise is resolved, and then it returns the result. It essentially allows you to write code that appears synchronous but behaves asynchronously.
This is the major difference between async and await in javascript.
3. Example 1: Fetching Data with async/await
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('Error fetching data:', error); } }
In this beginner level example, we use the fetch
API to make an HTTP request and await its response. The await
keyword allows us to pause the execution until the response is received. Using try-catch
, we handle any errors that might occur during the request.
4. Example 2: Chaining Multiple Async Functions
async function getProcessedData() { const rawData = await fetchData(); const processedData = await processRawData(rawData); return processedData; }
Here, we have two asynchronous functions explained, fetchData()
and processRawData()
. The ‘await’ keyword helps us chain these functions together easily, resulting in cleaner and more readable code.
5. Parallel Execution with async/await
async function getParallelData() { const [userData, productData] = await Promise.all([ fetchUserData(), fetchProductData() ]); console.log('User data:', userData); console.log('Product data:', productData); }
In this example, we use Promise.all()
with await
to fetch user and product data concurrently. This approach improves performance, as both requests are executed simultaneously.
6. Error Handling in async/await
async function performTask() { try { const result = await doSomethingAsync(); console.log('Task result:', result); } catch (error) { console.error('Error performing task:', error); } }
The try-catch block ensures that any errors thrown during asynchronous operations within the performTask()
function are caught and handled gracefully.
Conclusion
Async and await have revolutionized the way we handle asynchronous JavaScript, offering a more intuitive and elegant approach compared to traditional callbacks and promises.
By using async and await, developers can write cleaner, more organized, and easier-to-understand code, ultimately improving productivity and application performance.
As you continue to enhance your JavaScript skills, remember to leverage these powerful keywords to create efficient, maintainable, and future-proof code. Happy coding!