How to use Laravel 8 Custom Email Notification Template?
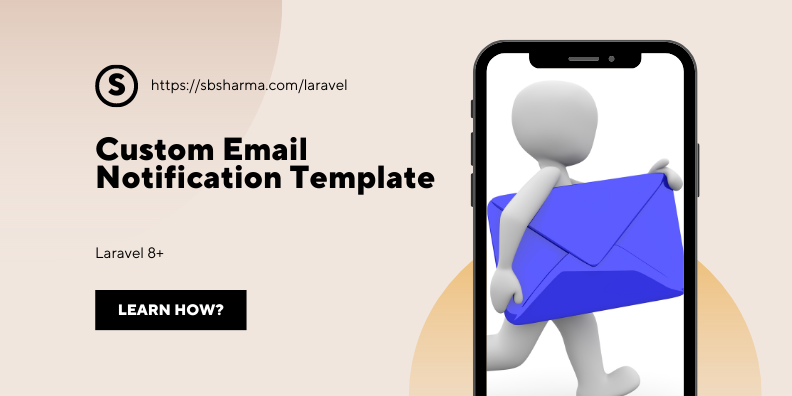
There are two ways to customize your laravel email notification template, let’s discuss them one by one.
- Customize the notification markdown template
- Use separate blade view html for email template of laravel notification
How to Customize the Laravel notification markdown?
By default laravel has a package to handle the notifications and the notification channel can be anything, like database, email, slack and many more.
The database notifications are used to show on the same platform, like I have a website and I want to show the notifications on the notification page to my user’s account.
Other notification channels are sending the data to the specified channel like email notification sends an email to the users.
There are some online tools to generate your expected markdown template by using WYSIWYG editors like stackEdit, Dillinger and onlinemarkdowneditor.dev etc.
Now, the fundamentals are clear, let’s start customizing the notification markdown template by following below steps.
1. Generate notification class
You need to generate the notification class first with the below command.
php artisan make:notification NewMessageNotification
The name of the notification class can be anything, there is no specific rule to choose the notification name but it can be something self-documented which describes its usage.
2. Publish the default notification resources
After generating the notification class, it’s time to publish the notification resources with the below artisan commands.
php artisan vendor:publish --tag=laravel-notifications
php artisan vendor:publish --tag=laravel-mail
The first command publishes the notification which copies the notification files from vendor to resources/views/vendor/notifications/email.blade.php
. This file only contains the final draft of the notification which means it uses the message, buttons and sub-copy components.
To get the full control over the template customization we need to run the second command. Which will copy the all blade files like buttons, header, footer, message and sub-copy to resources/views/vendor/mail/html
and to resources/views/vendor/mail/text
.
You can customize the css from resources/views/vendor/mail/html/themes/default.css file
.
Use html view blade file as a notification template
To use the blade file as a notification email template, you can use view()
method of the MailMessage
class. The blade can contain plain html or text or markdown as per your requirement. Just update the toMail()
method of your notification class as given below in the code.
public function toMail($notifiable) { return (new MailMessage) ->subject("New Notification") ->view('mail.template', [ 'content' => $this->content ]); }
As you can see in the code above, I am passing the content variable to the view template which I can use to fill the data with other html code. We have all required methods available on the MailMessage
class like, from, to, cc, bcc, subject and view etc.