Ionic 5 E-commerce Product Sorting and searching
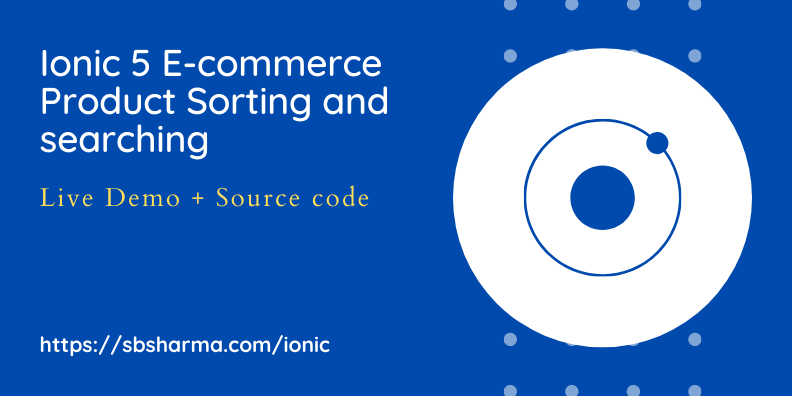
This is part 3 of the ionic 5 shopping app and we are going to add product sorting and searching in this app.
We will use following pages here
- Product listing page
- Product service page to apply sorting and searching
Our purpose is to maintain the sorting like when we filter the data it should be sorted as before.
Live Demo
Product sorting
Go to src/app/tab1
(Product listing page), we have added a sort icon before the filter icon and above the product listing. It will also ensure that if sorting is already applied, it will show a filled icon otherwise the outline icon will be there.
isSorted() { return Object.values(this.sort).some(el => el); }
When user click on the sort icon, it will open the ion-action-sheet with available sorting options:
- Latest products
- Price – Low to High
- Price – High to Low
After selecting one of the options from the above list, it will go to the product service applyLocalSort
method.
applyLocalSort ( column, order, type ) { this.uncheckSorts(); this.sort[type] = true; console.log('column :>> ', column); this.beforeSort = this.products; this.products = this.beforeSort.sort((a, b) => { console.log('sort :>> ', a, b); if ( order === 'desc' ) { return a[column] > b[column]; } else { return a[column] < b[column]; } }); this.showResultCount(); }
Product searching
Go to src/app/tab1
(Product listing page), we have already added an ion-searchbar there and now it is working.
<ion-searchbar mode="ios" (ionChange)="search($event)" debounce="500" style="padding: 16px 0px;"></ion-searchbar>
You can also integrate a common search page here but for this tutorial we are using this search box only.
On each search we are using product service method called searchProducts
with three product criteria,
- Name
- Category
- Price
The product searching will work in a sequence that if the searched term is not found in the name then it will move to category and then to price.
searchProducts( term: string ) { this.products = []; if ( `${term}`.trim() ) { let NotFoundInName = this.allProducts.map(item => { if ( item.name.toLocaleLowerCase().indexOf(term.toLocaleLowerCase()) < 0 ) { return item; } else { this.products.push(item); } }); console.log({NotFoundInName}); let NotFoundInCategory = NotFoundInName.map(item => { if (item) { if ( item.category.toLocaleLowerCase().indexOf(term.toLocaleLowerCase()) < 0 ) { return item; } else { this.products.push(item); } } }); console.log({NotFoundInCategory}); let foundInPrice = NotFoundInCategory.map(item => { if (item) { if ( `${item.price}`.toLocaleLowerCase().indexOf(term.toLocaleLowerCase()) > -1 ) { this.products.push(item); } } }); } else { this.products = this.allProducts; } this.showResultCount(); }
With this approach it will need to process less data, only the data which is not matched with searched Term.
Hope you enjoy the tutorial.
See you in the next learning journey.