How To Send Laravel Emails with PDF Attachment? (Examples)
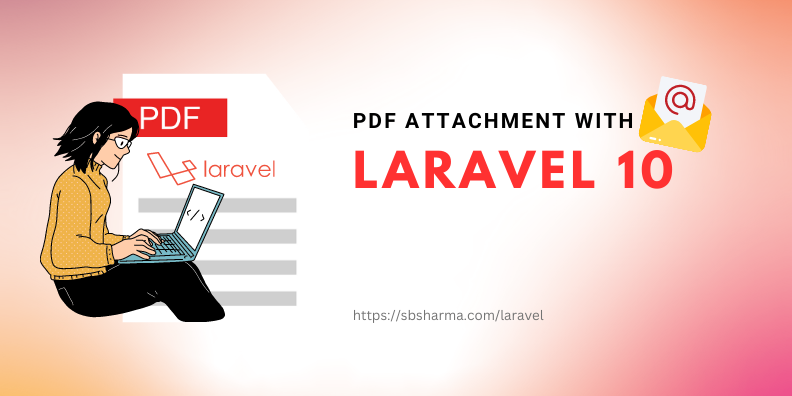
Sending PDF attachments via email is a common requirement in web applications. Laravel, being one of the most popular PHP frameworks, provides a simple and efficient way to achieve this. In this article, we will walk you through the process of sending PDF attachments using email in Laravel.
Setting up the Laravel Environment
The first step in sending PDF attachments using email in Laravel is to set up the environment. This involves installing and configuring Laravel, as well as any required libraries and packages. We assume that you have already set up Laravel and are familiar with its basic concepts. If not, please refer to the official Laravel documentation.
Install Laravel PDF Package
Before we can send PDF attachments via email, we need to generate the PDF files. Laravel provides several libraries for generating PDFs, such as Dompdf, Snappy, and TCPDF. In this article, we will be using Dompdf.
Dompdf is a PHP library that allows you to generate PDFs from HTML content. To install Dompdf in your Laravel application, you can use Composer:
composer require barryvdh/laravel-dompdf
Publish the PDF package resources
We can publish the package resources with the command below. It will copy the config file from vendor to laravel config/dompdf.php
directory. We can modify this file for PDF settings.
php artisan vendor:publish --provider="Barryvdh\DomPDF\ServiceProvider"
Setup mailtrap SMTP details
Before sending any email, we need to set up SMTP details in the env file.
MAIL_MAILER=smtp MAIL_HOST=smtp.mailtrap.io MAIL_PORT=2525 MAIL_USERNAME=234hjsdh234234 MAIL_PASSWORD=234hjsdh234234 MAIL_ENCRYPTION=tls MAIL_FROM_ADDRESS=example@email.com MAIL_FROM_NAME="domain"
Save the env file to update the SMTP details.
Email template
We have added the welcome email template to the mail view directory resources\views\mail\welcome.blade.php
Hi, {{ $user['name'] }},<br /> We are glad to welcome you here. Please find the attached letter.<br /> Best Regards,<br /> Xyz, Team <br />
Send Email with PDF attachment from Controller
Once the pdf package is installed, you can generate a PDF file from the laravel blade view file using the below code. Then Laravel provides a built-in mailer that makes it easy to send emails. To send an email with a PDF attachment, we can use the following code:
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Barryvdh\DomPDF\Facade\Pdf; use Illuminate\Support\Facades\Mail; class EmailController extends Controller { public function send() { $data = [ 'name' => 'Alex', 'position' => 'Head of content marketing', 'join_at' => '07/23' ]; $pdf = Pdf::loadView('pdf.letter', $data); $file = public_path("emails/welcome-letter.pdf"); $pdf->save($file); $this->send_mail($file); } public function send_mail($file) { $user = [ 'name' => 'alex', 'email' => 'alex@gmail.com' ]; Mail::send('mail.welcome', $user, function($message)use($user, $file) { $message->to($user['email']) ->subject('Welcome letter'); $message->attach($file); }); echo "Mail send successfully !!"; } }
This code generates a PDF file from the given blade template like pdf / letter.blade.php
And then store the pdf to the public directory, so that it can attach the pdf to the email notification.
Conclusion
In this article, we have shown you how to send PDF attachments using email in Laravel. We have covered the process of generating PDFs using the Dompdf library, and sending emails with PDF attachments using the built-in Laravel mailer. By following these steps, you can easily add PDF attachment functionality to your Laravel application.