Laravel redirects 7, 8 – with Examples
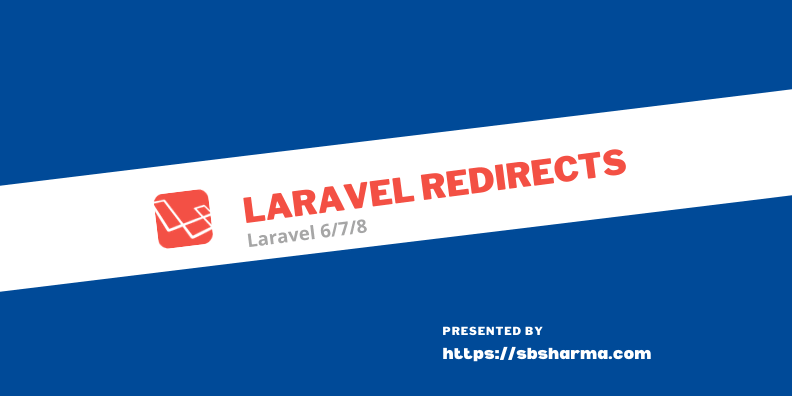
Today we are going to learn all about Laravel redirects with parameters.
Now, we have a simple global helper function that is available to use for laravel redirects with parameters or without parameters.
redirect( $to = null, $status = 302, $headers = [], $secure = null )
Redirect to a url with parameters
Example, Redirect to a url with parameters like shown below.
$tab = “general”; return redirect(“/settings/{$tab}”);
Then we can get this parameter into controller method.
public function settings( $tab ) { dd($tab); }
If you have a route name specified for this url, you can try Laravel redirects to a routes instead of urls.
Redirect to a route with parameters
Following example will help us to understand Laravel redirects in a better way.
Example 1.
Sometimes we want to redirect our response to a particular route but that route also needs a parameter. So, we can pass the parameter to a route like below
route( $route, $parameters = [], $status = 302, $headers = [] ) return redirect()->route(‘product’, [234]); // when url is : product/{id}
If we have multiple parameters we can pass them also as an associative array.
return redirect()->route(‘product.status’, [‘id’=>234, ‘status’ => ‘published’]); // when url is : product/{id}/{status}
Example 2.
Let’s create another example of Laravel redirects.
Route::get(‘settings/{tab}’, ‘User/SettingController@index)->name(‘settings.index’);
Now we can redirect to this route by just using the route name.
return redirect()->route(‘settings.index’, [‘tab’ => ‘general’]);
Redirect Back with parameters
Sometimes we want to redirect back to the last route. Then we can use the following way.
return redirect()->back();
Or
return back();
Well, this back method actually redirects to the previous route. So, If you have used parameters with the previous route it will work in the same way. You just returned back to that route and the rest process will go on like you navigate to that route.
Redirect back with flash data
Most of the time we want to show the success or failure message on the page after performing action.
Like when we delete an item we will get the response back and show the “successfully deleted” or “failed to delete” message on our page.
For those messages we can use flash session and flash session will automatically destroy the session after refresh the page.
return redirect()->back()->with([‘error’ => ‘Failed to delete!’]); return redirect()->back()->with([‘success’ => ‘Successfully deleted!’]); // And on the blade file we can use it like this @if (session(‘error’)) <div class="alert alert-danger"> {{ session(‘error’) }} </div> @endif @if (session(‘success’)) <div class="alert alert-‘success’"> {{ session(‘success’) }} </div> @endif
Redirect back with form data
Although, we have Laravel validation to manage form validation and auto redirect with form inputs and errors. But if you want to use it, you can redirect with inputs back to the input form.
return back()->withInput(); // withInput(?array $input = null)
We can also remove anything from the inputs array while returning it. Like below
return back()->withInput(Input::except(‘price’));
Now in the form all fields will be filled with values entered before submit the form but except the price input because we haven’t got that back.
Or we can also use this trick to send more parameters with the back method. Like example given below
return back()->withInput([‘one-parameter’ => ‘value’, ‘second-parameter’ => ‘value’ ]);
And we can use it on the page like
@if ( old(‘one-parameter’) ) // do something here @endif {{ old(‘second-parameter’) }}
Redirect back with form errors
Yes, we can also redirect back with form errors. Although we have a laravel validator for this, even then sometimes we need to return some custom errors back to the page.
return redirect()->back()->withErrors([‘country.required’, ‘Country is required']);
Redirect to a Laravel controller with parameters
This is one the useful redirect techniques because it allows us to call the controller methods directly without using any kind of route.
action( $action, $parameters = [], $status = 302, $headers = [] ) return redirect()->action([GeneralSettingsController::class, 'index']);
Or if we need to pass the parameter to a method, we can use following way
return redirect()->action([ProductsController::class, ’show’], [‘id’ => 24]);
Redirect to an external url to the next tab
Few days ago I needed this. So, I used this system to create the anchor html tag with target _blank. Like below
<a href=”{{ route(‘goToExternalUrl’, [‘external-url.com’]) }}” target=”_blank”>open it</a>
And in the controller method
openExternalUrl ($url) { // do so manipulation here and prepare the code to get the return redirect($url); }
It will open the url to the next tab. If you know another way to achieve this plz don’t forget to comment here.
Hope you find this article useful. Happy LaraCode.