Mailtrap Integration for Email Testing with Laravel 10
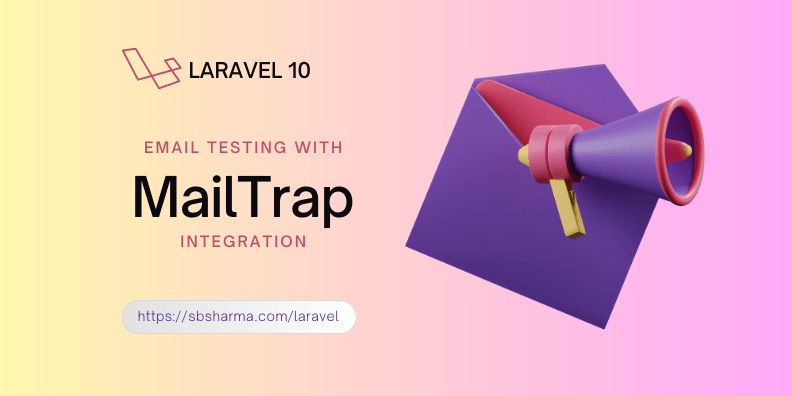
Today we will discuss about the Mailtrap integration with laravel 10 .
Sending and receiving emails is a fundamental aspect of modern web applications, allowing communication between users and providing essential notifications.
However, during development, it can be challenging to test email functionality without sending real emails to actual recipients. This is where Mailtrap comes to the rescue.
In this article, we will explore how to integrate Mailtrap with Laravel 10 for effective email testing.
What is Mailtrap?
Mailtrap is a popular email testing tool that provides a simulated SMTP server to capture and display emails sent from development environments. It allows developers to test email sending functionality without delivering emails to real recipients. Instead, the emails are captured and displayed within the Mailtrap dashboard. It is a valuable tool for quality assurance and debugging.
Read also: How to integrate mailchimp APIs with laravel 10?
Prerequisites
Before we begin, make sure you have the following set up:
- Laravel 10 installed on your development machine.
- A Mailtrap account. You can sign up for free at mailtrap.com.
Step 1: Configure Mailtrap in Laravel
First, let’s configure Laravel to use the Mailtrap SMTP server for sending emails.
Open the .env
file in the root of your Laravel project and add the following lines:
MAIL_MAILER=smtp MAIL_HOST=smtp.mailtrap.io MAIL_PORT=2525 MAIL_USERNAME=your_mailtrap_username MAIL_PASSWORD=your_mailtrap_password MAIL_ENCRYPTION=tls
Replace your_mailtrap_username
and your_mailtrap_password
with your actual Mailtrap credentials.
Step 2: Sending Test Emails to test the mailtrap integration with laravel 10
Now, let’s send a test email using Laravel’s built-in email functionality. We’ll create a route and a controller method to trigger the sending of the test email.
In your routes/web.php
file, add the following route:
use App\Http\Controllers\MailController; Route::get('/send-test-email', [MailController::class, 'sendTestEmail']);
Next, create a new controller named MailController
by running the following command:
php artisan make:controller MailController
Now, open the MailController.php
file located in the app/Http/Controllers
directory and add the following code to the sendTestEmail
method:
use Illuminate\Support\Facades\Mail; use App\Mail\TestMail; public function sendTestEmail() { Mail::to('recipient@example.com')->send(new TestMail()); return "Test email sent!"; }
In this example, we assume you have a TestMail
class defined in the app/Mail
directory. If not, create one using the following command:
php artisan make:mail TestMail
Open the TestMail.php
file located in the app/Mail
directory and modify the build method to define the email content:
public function build() { return $this->subject('Test Email')->view('emails.test'); }
Create a new Blade view file named test.blade.php
in the resources/views/emails
directory and add your test email content, such as:
<p>This is a test email sent from Laravel using Mailtrap!</p>
Step 3: Viewing Emails in Mailtrap
With the test email sending functionality in place, it’s time to check Mailtrap to see if the email is captured and displayed.
- Log in to your Mailtrap account.
- From the dashboard, select your inbox or create a new one.
- You will find the test email you sent listed in the inbox. Click on it to view the email content.
Congratulations! You’ve successfully integrated Mailtrap with Laravel 10 for email testing.
FAQ and Common Issues
1. Why am I not receiving emails in Mailtrap?
Ensure that your Mailtrap credentials in the .env file are correct. Double-check the MAIL_USERNAME and MAIL_PASSWORD values. Also, make sure you are sending emails to an address associated with your Mailtrap inbox.
2. Why is the email content not displayed correctly in Mailtrap?
Ensure that the view used in the build method of your mail class (TestMail in this example) matches the actual path to your Blade view file.
3. Can I use Mailtrap for testing other email-related features?
Yes, Mailtrap can be used to test other email-related features such as attachments, HTML formatting, and email notifications triggered by different events in your Laravel application.
4. Is Mailtrap only for Laravel?
No, Mailtrap can be integrated with various programming languages and frameworks. It provides SMTP server details that can be used in any application that sends emails.
Conclusion
Mailtrap is an invaluable tool for testing email functionality during Laravel application development. With the Mailtrap integration with Laravel 10, you can confidently test your email sending features without worrying about spamming real recipients.
This article guided you through the process of setting up Mailtrap, sending test emails, and viewing them within the Mailtrap dashboard. With this knowledge, you can enhance the quality and reliability of your email notifications in Laravel applications.