Open File To The New Tab Using JavaScript Blob URL
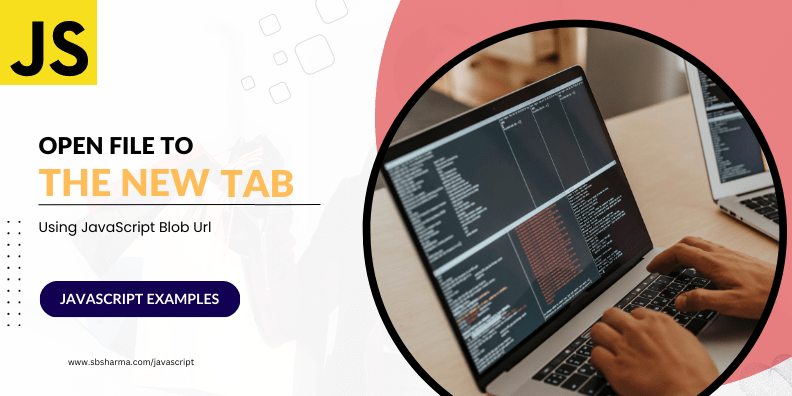
JavaScript is a versatile programming language that allows developers to create dynamic and interactive web pages. One common task in web development is displaying PDF documents and images to users. While there are several ways to achieve this, one effective approach is to use Blob URLs to open and view PDF and image files in a new tab. In this article, we will explore how to accomplish this using JavaScript by uploading files from an HTML form.
What is a Blob URL?
A Blob (Binary Large Object) is a data type that represents arbitrary data, such as multimedia files, in a single file-like object. Blob URLs, also known as Object URLs, are unique references to blobs stored in memory. They provide a way to access and handle binary data directly in the browser without the need for a server request.
Generating a Blob URL from an HTML Form
To open a PDF document or an image file in a new tab using a Blob URL, we first need to generate the Blob object from the file uploaded through an HTML form. Let’s take a look at the steps involved:
Step 1. Handle the File Upload
In your HTML file, create a form with an input field of type file to allow users to select a file for upload:
<form id="fileForm"> <input type="file" id="fileInput"> <button type="submit">Upload</button> </form>
Step 2. Handle the Form Submission
Next, we need to handle the form submission in JavaScript. Add an event listener to the form’s submit event, and prevent the default form submission behavior:
const fileForm = document.getElementById('fileForm'); fileForm.addEventListener('submit', function(event) { event.preventDefault(); // Process the uploaded file here });
Step 3. Process the Uploaded File
Inside the form submission handler, we can access the uploaded file using the files property of the file input element. To generate a Blob object from the uploaded file, we can use the Blob()
constructor. Here’s an example:
const fileInput = document.getElementById('fileInput'); const uploadedFile = fileInput.files[0]; const blobData = new Blob([uploadedFile], { type: uploadedFile.type });
The above code creates a Blob object from the uploaded file, preserving its original file type.
Step 4. Create a Blob URL
Once we have the Blob object, we can create a URL for it using the URL.createObjectURL()
method. This method takes the Blob as an argument and returns a unique URL that can be used to access the blob data.
const blobUrl = URL.createObjectURL(blobData);
Step 5. Opening the Blob URL in a New Tab
Now that we have the Blob URL, we can open it in a new browser tab. To achieve this, we can use the window.open()
method, passing the Blob URL as the URL argument.
window.open(blobUrl, '_blank');
The above code will open the Blob URL in a new tab or window, depending on the user’s browser settings.
Step 6. Releasing the Blob URL
After opening the Blob URL, it’s important to release the memory associated with it to prevent memory leaks. We can do this by revoking the URL using the URL.revokeObjectURL()
method.
URL.revokeObjectURL(blobUrl);
By calling this method, we ensure that the memory resources allocated for the Blob URL are freed up. I have also written an article on URL and parameter binding in JavaScript, you can check that.
Example: Opening a PDF in a New Tab
Let’s put the steps together and see an example of opening a PDF document in a new tab after uploading it through an HTML form:
const fileForm = document.getElementById('fileForm'); fileForm.addEventListener('submit', function(event) { event.preventDefault(); const fileInput = document.getElementById('fileInput'); const uploadedFile = fileInput.files[0]; const blobData = new Blob([uploadedFile], { type: uploadedFile.type }); const blobUrl = URL.createObjectURL(blobData); window.open(blobUrl, '_blank'); URL.revokeObjectURL(blobUrl); });
In the above example, we handle the form submission event. Then retrieve the uploaded file, create the Blob object and create the Blob URL. After blob URL, we can open it in a new tab, and finally release the URL.
Conclusion
Using Blob URLs in JavaScript provides an efficient and straightforward way to open and view PDF documents and images in a new tab. By uploading the file from an HTML form, creating a Blob URL, and opening it with window.open()
. We can enhance the user experience and provide a seamless way to view PDFs and images in a new tab.