PHP variables with a string literal
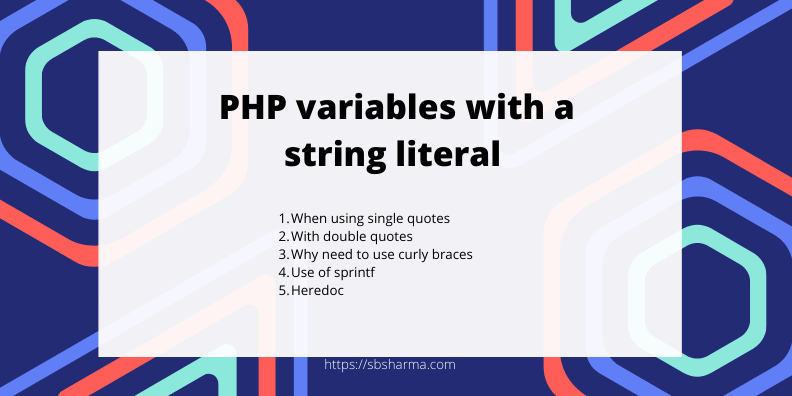
While working with PHP, most of the time we need to use PHP string literal variables. Then we use string concatenation techniques.
PHP String literal variable
PHP string literal variable is a technique to use the variable with text or string to make text preview dynamic. Every time your variable value will change, it will effect the text and then you will get the dynamic content.
But there are few more methods to render the PHP variable value inside the string literal.
- Use single quotes
- Use double quotes
- Use curly braces
- Use of sprintf
- Heredoc
Read also : Create, modify and update excel sheet with PHP library
When using single quotes
We can use single quotes for variable interpolation with string or PHP string literal variable. For this we need to use a concatenation operator to concat string with variable.
<?php //phpinfo(); $name = "harry"; $subject = 5; $total_marks = 500; $obtained_marks = 423; $exam_cycle = 'month'; // string concatenation with single quotes echo 'Your name is '.$name.'!';
With double quotes
We can use double quotes for variable interpolation and this time we don’t need any concatenation operator. Double quotes allow us to render the value of the variable inside the string without using any other operator.
// dobule quotes echo "<br>and your got the marks $obtained_marks";
When we need to use curly braces with double quotes
Sometimes, you heard about curly braces with double quotes to use PHP string literal variable. But I was surprised when I heard about it for the first time because I am easily able to use variables with double quotes. Then why do we need to use curly braces?
Then I found the case where we need to use curly braces. Let’s suppose we are going to print a fees cycle for students Like this monthly, quarterly or yearly.
And we have variables with value “month, quarter or year” then we need variable interpolation without space with string to get the desired result.
This is the situation where we need to use curly braces. Curly braces separate the variable from the other string.
// use of curly braces with double quotes echo "<br>Your exam cycle is {$exam_cycle}ly";
Use of Sprintf
Sprintf allows us to use complex variable expressions easily. For example we are calculating average marks here and print with the help of sprintf.
Sprintf simply uses the spots where the variables can be placed with %s syntax.
// sprintf echo sprintf("<br>and your avg marks is %s", $obtained_marks/$total_marks*100); echo sprintf("<br>You got %s out of %s", $obtained_marks, $total_marks);
Heredoc
One more way to use variables with string is heredoc. The syntax of heredoc is <<< then use the identifier and press enter to use the new line.
Here we can use our string and then the same identifier will be used to close the heredoc.
Closing identifier must be in the new line. Identifiers must be alphanumeric and must start with a non-digit character or underscore.
Example of using heredoc. Hope you can relate with the example below.
echo <<<STR <div class="container"> <p style="font-size:12px;line-height:1.5px;"> <span class="first-item" style="color:red">Hello world</span> <span class="last-item" style="color:black">Lore ipsume</span> </p> </div> STR;
You have learnt about the use of PHP string literal variable.
Hope you find this article useful. Keep learning, stay inspired
See you in the next learning chapter.