How to return the response from an asynchronous call in javascript?
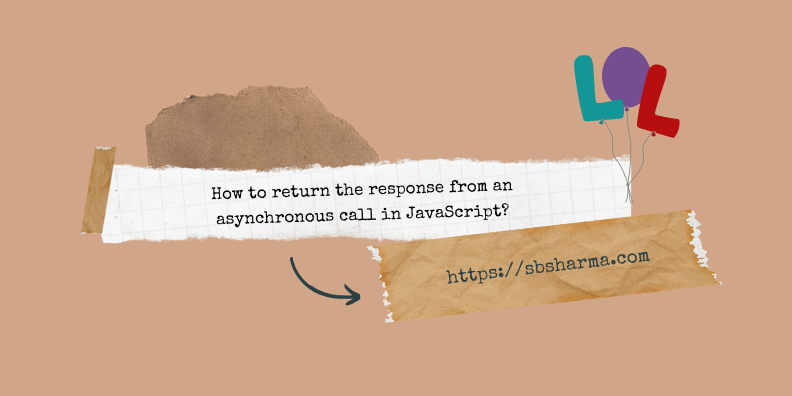
Return the response from an asynchronous call can be frustrating for javascript beginners. Once in a while Javascript developers are stuck at this point.
First of all javascript is a frontend programming language and if we have some ajax calls in the code then these can interrupt the page loading. That’s why these requests are asynchronous calls and work parallel to the rest of the page rendering.
Where does an asynchronous call create the problem?
Look at the below example, where it can create the problem to return the response from an asynchronous call.
function _getImages(per_page) { var result; fetch( `https://pixabay.com/api/?key=${api_key}&q=${ query }&image_type=""&per_page=${per_page}&page=${page++}` ).then(response => { result = response; }) return result; } var get_images = _getImages(4); console.log('Plain asynchronous call >>', {get_images});

Actually, it loads the console output before the Ajax call finishes and return the response from an asynchronous call. This is asynchronous behavior.
How to deal with asynchronous functions without compromise with their default features?
- Callback function
- Promise
- Async and await
Callback function
We can pass the callback function to ajax request and it will return the response to that callback function. Callback functions are popular in the node.js.
function _getImages(per_page, callback) { const response = fetch( `https://pixabay.com/api/?key=${api_key}&q=${ query }&image_type=""&per_page=${per_page}&page=${page++}` ); response.then(callback) } function _loadImagesToGallery(imgObj) { imgObj.json().then(res => { console.log('using callback >> ', res.hits); }) } _getImages(4, _loadImagesToGallery);
JavaScript Promises
JavaScript Promises was introduced in ES2015.
We can use a JavaScript promise with ajax call and it will resolve or reject the response. It returns the promise object synchronously and then we can get the response by using then
and catch
.
In other words, promises just add the listener to asynchronous calls and when it gets the value it return the response from an asynchronous call.
function promise_getImages(per_page) { return new Promise((resolve, reject) => { const response = fetch( `https://pixabay.com/api/?key=${api_key}&q=${ query }&image_type=""&per_page=${per_page}&page=${page}` ); response.then(res => { try { resolve(res.json()) } catch(error) { reject(error) } }) }) } promise_getImages(4).then(res => { console.log('using promise >>', res.hits); })
Async and await
Back in 2017, JavaScript added async functions and the await keyword.
It is the same as the javascript promise but it has some better syntax and needs less code. Async and await look like synchronous calls and it provides a better understandable code view.
async function getImages(per_page) { const response = await fetch( `https://pixabay.com/api/?key=${api_key}&q=${ query }&image_type=""&per_page=${per_page}&page=${page++}` ); const data = await response.json(); return data.hits; } function loadImagesToGallery(per_page) { getImages(per_page).then(imgObj => { console.log(‘async and await >> ’, imgObj); }) } loadImagesToGallery(4);
Ref: Return the response from an asynchronous call in JavaScript
We have created an image gallery with jQuery and have used pixabay API to load the images, you can check the article here with live demo of image gallery. All the source code also available on the codepen.
Hope you find this article useful, If so please share it with your friends. Don’t forget to comment your suggestions or queries. It will help us to grow together.