How to add custom error message to the error bag without validation in Laravel 8.x?
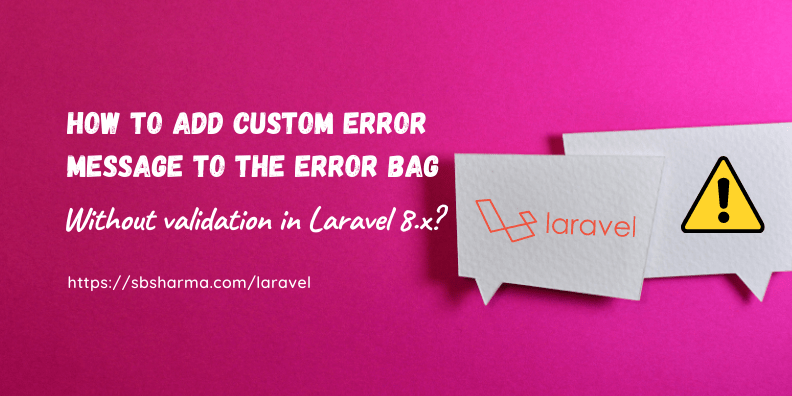
Often we need to add a custom error message to the error bag without validation in our laravel application.
So, today we are going to discuss this scenario in depth. Let’s get started.
Add error message to the Laravel message bag
If you have a custom error message that you want to inject into Laravel’s error bag, you can do so by using the Laravel MessageBag
class.
Here’s an example of how you can achieve this:
Example of message bag in Laravel
use Illuminate\Support\MessageBag; public function store(Request $request) { $errors = new MessageBag(); $errors->add('custom_key', 'Your custom error message goes here'); return redirect()->back()->withErrors($errors)->withInput(); }
In the above example, we create a new instance of the MessageBag
class and add a custom error message to it using the add()
method. The first parameter of the add()
method is the key name you want to assign to the error message, and the second parameter is the error message itself.
Then, we redirect back to the previous page with the errors injected into the error bag using the withErrors($errors)
method. The withInput()
method is used to repopulate the form fields with the previously entered values.
Use of custom error in the blade view file
In your view, you can access the custom error message using the assigned key name:
<!-- View file --> @error(‘custom_key’) <div class="alert alert-danger">{{ $message }}</div> @enderror
By following this approach, you can inject a custom error message with a specific key name into Laravel’s error bag and display it in your views.
That’s it, See you in the next Laravel hack, tips & tricks.